Welcome to Module 1 – ( Introduction to Pandas ) of our comprehensive Pandas tutorial series! In this module, we’ll introduce you to the world of Pandas, a powerful Python library designed for data manipulation and analysis. By the end of this module, you’ll understand what Pandas is, why it’s crucial for data analysis, how to install it, and the core Pandas data structures: Series and DataFrame.
1. What is Pandas?
Definition
Pandas is an open-source Python library that provides easy-to-use data structures and data analysis tools. It was created by Wes McKinney in 2008 and has since become an essential tool for data scientists, analysts, and researchers.
Why Pandas?
Simplifies Data Handling: Pandas simplifies the process of working with structured data, making it easier to clean, transform, and analyze data.
Key Features:
- Efficient data manipulation with DataFrame and Series.
- Powerful data alignment and indexing.
- Tools for reading and writing data from various file formats.
- Data cleaning and preprocessing capabilities.
Use Cases:
- Data cleaning and preparation.
- Data analysis and exploration.
- Time series data analysis.
- Data visualization and reporting.
2. Why use Pandas?
Data Challenges
Data analysis often involves dealing with messy and complex datasets. Common data challenges include:
- Missing data.
- Merging data from multiple sources.
- Filtering and selecting specific data.
- Aggregating and summarizing data.
- Reshaping data for analysis.
- Working with time series data.
Key Features of Pandas
Pandas addresses these challenges with its key features:
Data Structures:
- Series: One-dimensional labeled arrays.
- DataFrame: Two-dimensional labeled data structures (tables).
Data Alignment:
- Automatically aligns data based on labels.
Handling Missing Data:
- Provides tools for detecting and dealing with missing data.
Data Aggregation:
- Easily aggregates data using grouping operations.
Data Transformation:
- Supports data cleaning, filtering, and transformation.
3. Installation and Importing Pandas
Installation
You can install Pandas using pip
or conda
:
# Using pip
pip install pandas
# Using conda
conda install pandas
Importing Pandas
Once installed, you can import Pandas into your Python script or Jupyter Notebook:
import pandas as pd
Now you’re ready to use Pandas in your data analysis projects!
Check the Pandas version installed
import pandas as pd
print(pd.__version__)
Output :


4. Pandas Data Structures
Pandas introduces two primary data structures:
Series: Introduction and Creation1
What is a Series?
A Series is a one-dimensional labeled array capable of holding various data types. It’s like a column in a spreadsheet or a single column of data in a DataFrame.
Creating a Series
You can create a Series using Pandas from various data sources, including lists, arrays, or dictionaries. For example:
import pandas as pd
data = [1, 2, 3, 4, 5]
series = pd.Series(data)
print(series)
Output:
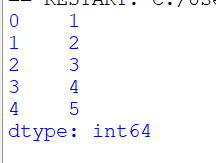
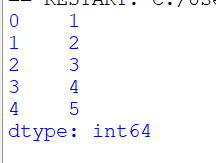
Series vs. Python Lists
Series offer advantages over Python lists, such as built-in labels and enhanced data manipulation capabilities.
DataFrame: Introduction and Creation2
What is a DataFrame?
A DataFrame is a two-dimensional, size-mutable, and heterogeneous tabular data structure with labeled axes (rows and columns). It’s like a spreadsheet or SQL table.
Creating a DataFrame
DataFrames can be created from various data sources, such as dictionaries, lists of dictionaries, or external data files like CSV and Excel.
Here’s an example of creating a DataFrame from a dictionary:
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df)
Output:


Differences between Series and DataFrame
Series are one-dimensional, while DataFrames are two-dimensional. DataFrames are used for more complex data analysis tasks, while Series are suitable for simpler tasks.
Congratulations! You’ve completed Module 1 of our Pandas tutorial. In this module, you’ve learned what Pandas is, why it’s essential for data analysis, how to install it, and the basics of Pandas data structures: Series and DataFrame.
In the next module, we’ll dive deeper into basic data manipulation with Pandas, including loading data, data exploration, indexing, and selection. Get ready to enhance your data analysis skills further!
One Comment