We’ll use Python and Flask, pythons pytube library to build a web-based YouTube video downloader in this tutorial. With just the URLs of the YouTube videos, we can interactively retrieve and download them thanks to this combination.
First, let’s look at the HTML (index.html) structure that serves as the basis for our webpage. The form on this simple HTML layout allows users to enter the URL of the video they wish to download.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Video Downloader</title>
</head>
<body>
<h1>Video Downloader</h1>
<form action="/download" method="post">
<label for="video_url">Enter video URL:</label>
<input type="text" id="video_url" name="video_url">
<input type="submit" value="Download">
</form>
</body>
</html>
The Flask framework and the Python script creates the backend functionality needed to manage the video download procedure.
from flask import Flask, render_template, request
from pytube import YouTube
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/download', methods=['POST'])
def download():
video_url = request.form['video_url']
try:
yt = YouTube(video_url)
video = yt.streams.get_highest_resolution()
download_path = 'path_to_save_video' # Specify your download path
video.download(download_path)
return "Download complete!"
except Exception as e:
return "An error occurred: " + str(e)
if __name__ == '__main__':
app.run(debug=True)
We’ve developed a straightforward but effective YouTube video downloader by combining HTML for the front-end structure, Python with Flask for server-side scripting, and the pytube library to manage YouTube video downloads. The video URL can be entered by users, starting the download process from the specified webpage.
The terms of service on YouTube and the rights of content creators must be respected when using this tool. Securing adherence to ethical and legal standards, this project can be used as a foundation for more advanced video downloader applications.
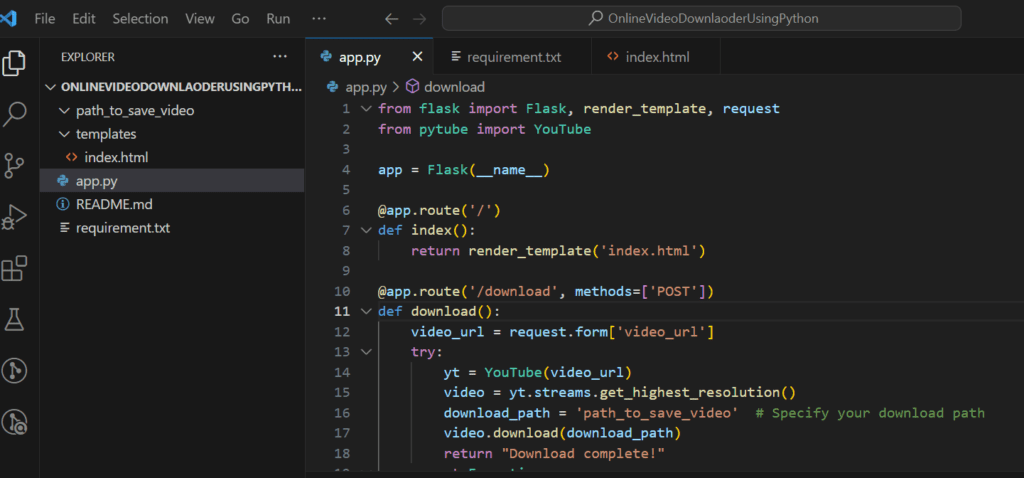
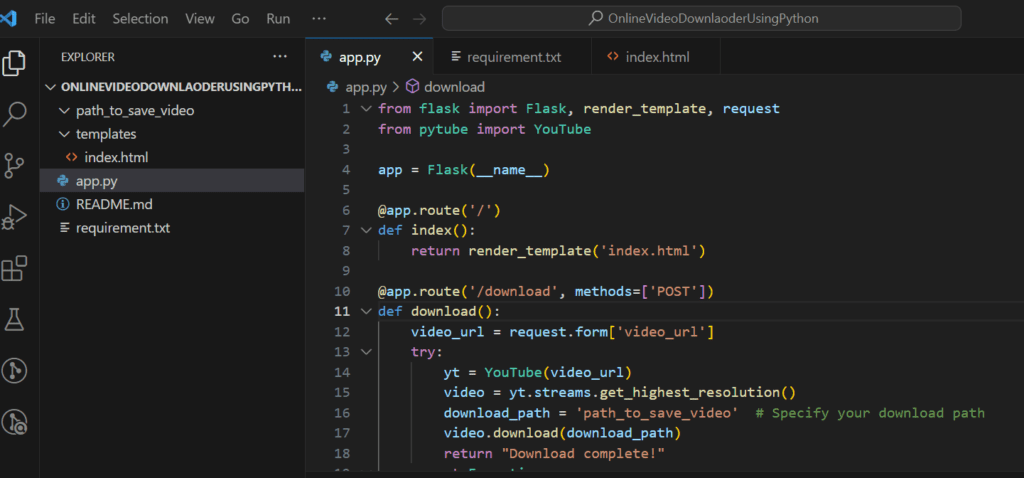
Feel free to build upon this foundation, adding features and tailoring it to particular requirements, all the while adhering to the terms of the platforms where the videos are being downloaded.