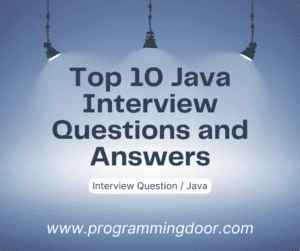
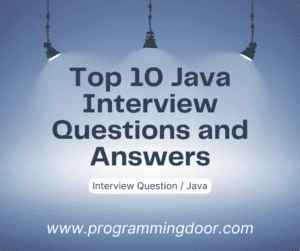
- What is Java?
- Java is a high-level, object-oriented programming language that is widely used to develop desktop applications, web applications, mobile applications, and enterprise software. It was created by James Gosling and his team at Sun Microsystems and was released in 1995.
- What are the key features of Java?
- Some of the key features of Java include platform independence, object-oriented programming, memory management, robustness, security, and multithreading.
- What is the difference between an abstract class and an interface in Java?
- An abstract class is a class that cannot be instantiated and can have abstract methods as well as concrete methods. An interface, on the other hand, is a collection of abstract methods that can be implemented by any class. An interface cannot have any concrete methods.
- What is the difference between the stack and the heap in Java?
- The stack is used to store primitive data types and references to objects, while the heap is used to store objects and their instance variables. The stack is limited in size and is managed automatically by the JVM, while the heap can grow dynamically based on the memory requirements of the program.
- What is the difference between a checked and an unchecked exception in Java?
- A checked exception is an exception that is checked at compile time, which means that the code must either catch the exception or declare it in a throws clause. An unchecked exception, on the other hand, is an exception that is not checked at compile time and can be caught or not caught by the code.
- What is the difference between a class and an object in Java?
- A class is a blueprint or template that defines the properties and methods of an object, while an object is an instance of a class that has its own state and behavior.
- What is the purpose of the static keyword in Java?
- The static keyword is used to define a class-level variable or method that can be accessed without creating an instance of the class. Static variables and methods are shared by all instances of the class.
- What is the purpose of the final keyword in Java?
- The final keyword is used to define a variable or method that cannot be changed or overridden. A final variable is a constant, while a final method cannot be overridden by a subclass.
- What is the purpose of the synchronized keyword in Java?
- The synchronized keyword is used to define a block of code that can only be accessed by one thread at a time. This is used to prevent concurrent access to shared resources and to ensure thread safety.
- What is the difference between an ArrayList and a LinkedList in Java?
- An ArrayList is implemented as an array and provides constant-time access to individual elements. A LinkedList, on the other hand, is implemented as a sequence of nodes and provides constant-time insertion and deletion of elements. However, accessing individual elements in a LinkedList takes linear time.