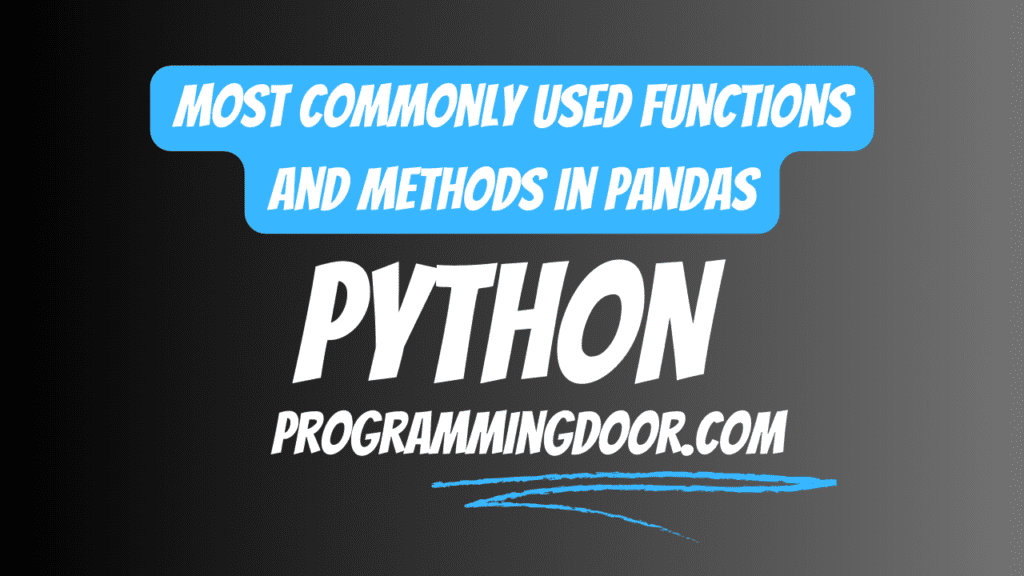
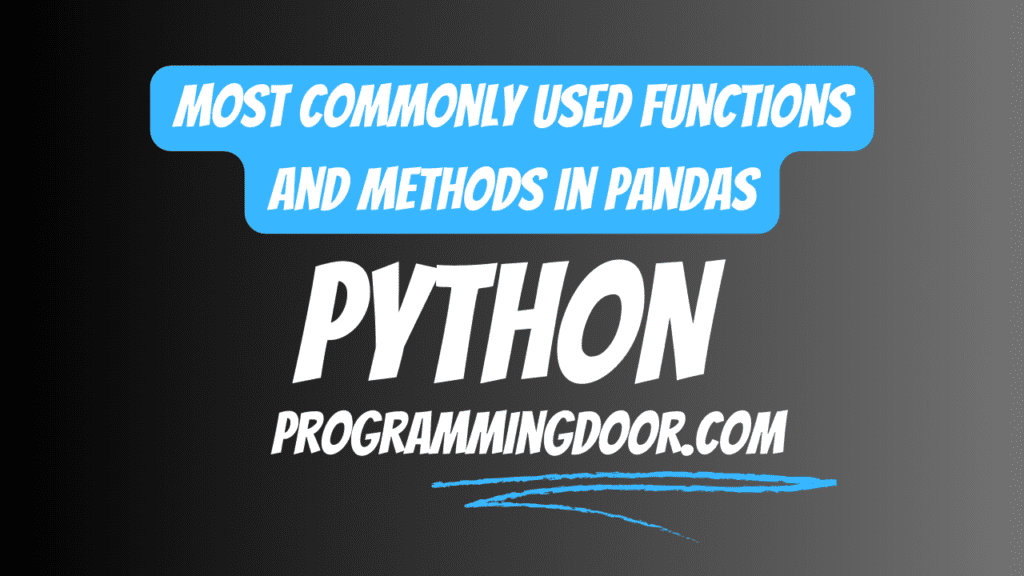
Pandas is a powerful library for data manipulation and analysis in Python. While it offers a wide range of functions and methods, listing all of them here would be impractical. Instead, I’ll provide an overview of some of the most commonly used functions and methods in Pandas:
Data Structures:
pd.DataFrame()
: Creates a DataFrame, the primary data structure in Pandas.pd.Series()
: Creates a Series, a one-dimensional labeled array.
Reading and Writing Data:
pd.read_csv()
: Reads data from a CSV file and creates a DataFrame.pd.read_excel()
: Reads data from an Excel file and creates a DataFrame.df.to_csv()
: Writes a DataFrame to a CSV file.df.to_excel()
: Writes a DataFrame to an Excel file.
Data Exploration:
df.head()
: Returns the first n rows of the DataFrame.df.tail()
: Returns the last n rows of the DataFrame.df.info()
: Provides information about the DataFrame, including data types and missing values.df.describe()
: Generates summary statistics for numerical columns.df.shape
: Returns the dimensions of the DataFrame (rows, columns).df.columns
: Returns a list of column names.df.dtypes
: Returns the data types of each column.
Data Selection and Indexing:
df['Column_Name']
: Accesses a specific column by name.df.loc[]
: Selects rows and columns by labels.df.iloc[]
: Selects rows and columns by integer positions.df.at[]
: Accesses a single cell by label.df.iat[]
: Accesses a single cell by integer position.
Data Manipulation:
df.sort_values()
: Sorts the DataFrame by one or more columns.df.groupby()
: Groups data for aggregation.df.drop()
: Drops specified rows or columns.df.rename()
: Renames columns.df.pivot_table()
: Creates a pivot table for data summarization.df.merge()
: Combines DataFrames through SQL-style joins.df.concat()
: Concatenates DataFrames vertically or horizontally.
Data Cleaning:
df.fillna()
: Fills missing values with specified values.df.dropna()
: Drops rows with missing values.df.replace()
: Replaces specified values with other values.df.duplicated()
: Checks for duplicate rows.df.drop_duplicates()
: Drops duplicate rows.
Statistical Functions:
df.mean()
: Calculates the mean of each column.df.median()
: Calculates the median of each column.df.sum()
: Calculates the sum of each column.df.min()
: Finds the minimum value in each column.df.max()
: Finds the maximum value in each column.
Visualization:
df.plot()
: Creates various types of plots from DataFrame data.df.hist()
: Generates histograms for numerical columns.
Time Series:
pd.to_datetime()
: Converts a column to datetime format.df.resample()
: Aggregates time series data.
Merging and Joining:
pd.concat()
: Concatenates DataFrames.df.merge()
: Merges DataFrames using SQL-style joins.
This list provides an overview of some of the most commonly used functions and methods in Pandas. The library offers many more functions and methods for advanced data manipulation, analysis, and transformation, making it a powerful tool for data scientists and analysts.