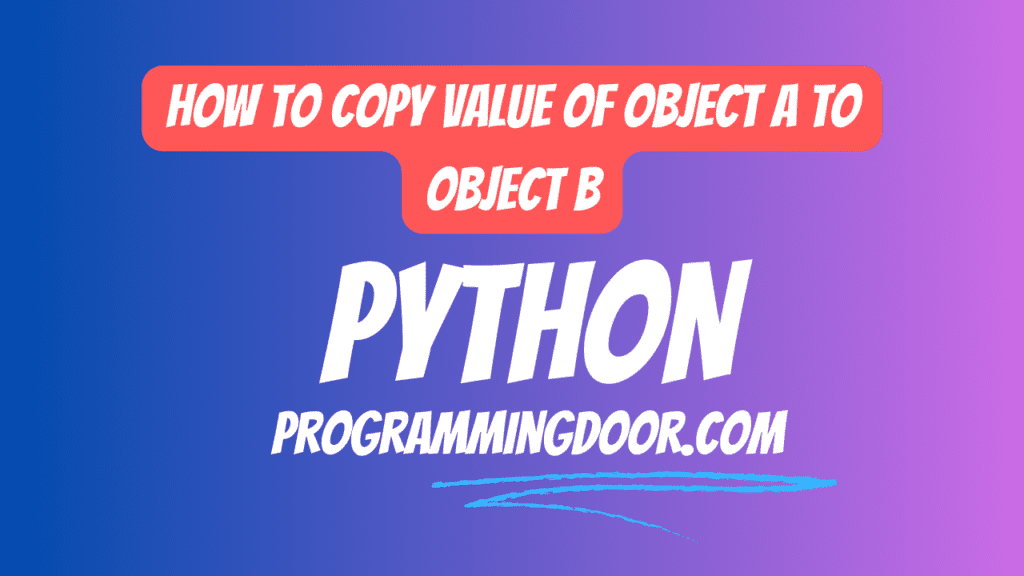
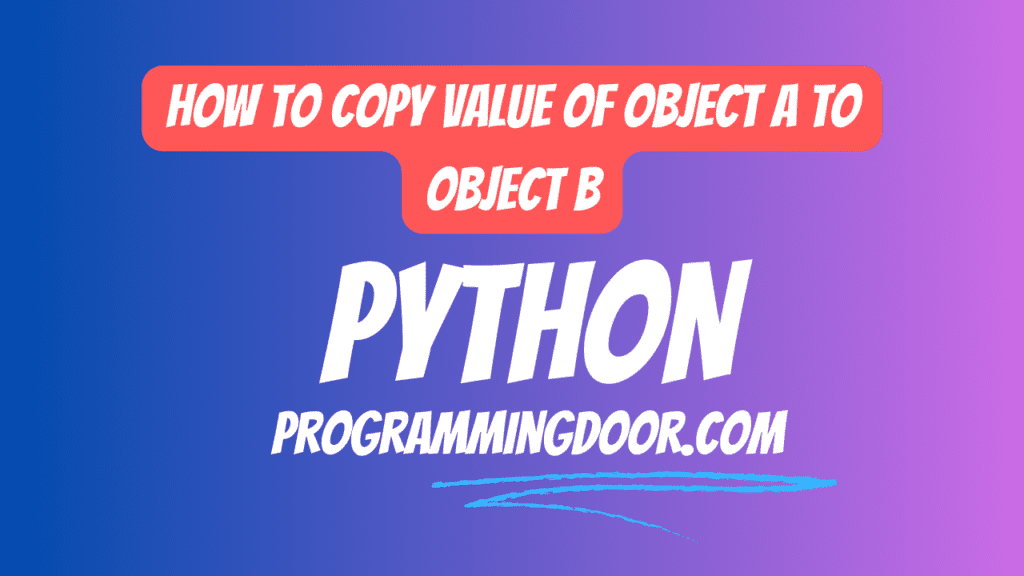
To copy the values of one object (let’s call it Object A) to another object (Object B) in Python, you have several options depending on the type of objects you’re working with. Here are some common methods:
- Using Assignment: If Object A is a simple data type (e.g., integers, strings) or an immutable object (e.g., tuples), you can simply assign its value to Object B.
a = 42
b = a # Copy the value of 'a' to 'b'
This method creates a new variable (Object B) with the same value as Object A.
- Using Copy Method (for Mutable Objects): If Object A is a mutable object like a list or a dictionary, you can use the
copy()
method or thelist()
ordict()
constructor to create a shallow copy of Object A and assign it to Object B. A shallow copy means that Object B will contain references to the same objects as Object A.
list_a = [1, 2, 3]
list_b = list_a.copy() # Shallow copy
Now, changes to list_a
won’t affect list_b
, and vice versa.
- Using Import (for Custom Objects): If Object A is an instance of a custom class, you can use the
copy
module to perform a shallow copy or deep copy depending on your needs.
import copy
class MyClass:
def __init__(self, value):
self.value = value
obj_a = MyClass(42)
obj_b = copy.copy(obj_a) # Shallow copy
obj_c = copy.deepcopy(obj_a) # Deep copy (for nested objects)
Shallow copy creates a new instance of the class, but the internal objects may still be shared, while deep copy creates entirely new objects.
The method we choose depends on the type of objects we are working with and whether we need a shallow or deep copy. Remember that for mutable objects like lists and dictionaries, it’s crucial to use a method that creates a copy to avoid unintended side effects when modifying the objects.