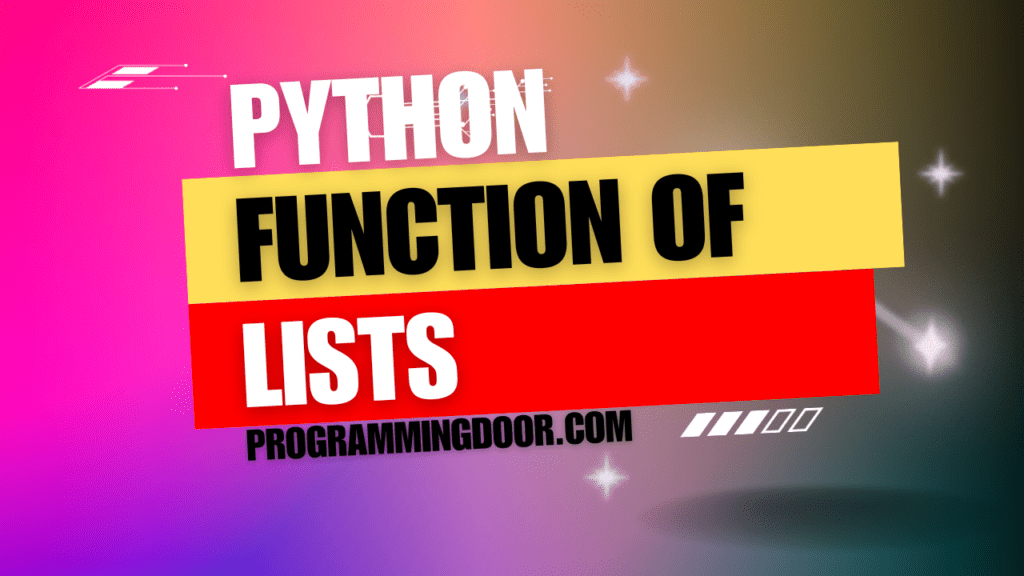
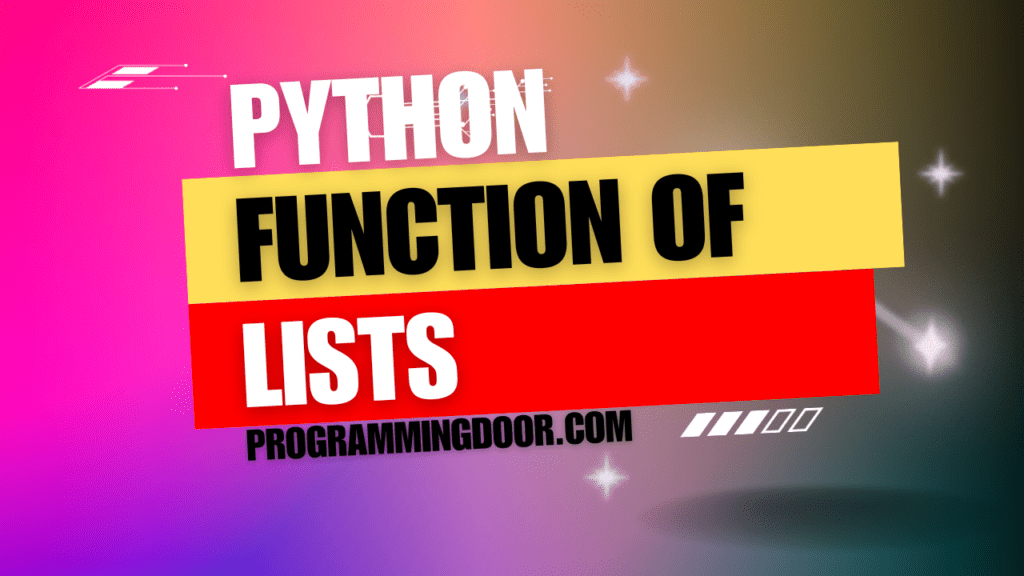
Lists in Python are versatile data structures that come with a variety of built-in functions and methods. These functions and methods allow you to manipulate and work with lists efficiently. Here are some of the most commonly used functions and methods for lists:
1. len()
: Returns the number of items in a list.
my_list = [1, 2, 3, 4, 5]
length = len(my_list) # Returns 5
2. append()
: Adds an item to the end of the list.
my_list = [1, 2, 3]
my_list.append(4) # Appends 4 to the end of the list
3. insert()
: Inserts an item at a specific position in the list.
my_list = [1, 2, 4]
my_list.insert(2, 3) # Inserts 3 at index 2, the list becomes [1, 2, 3, 4]
4. remove()
: Removes the first occurrence of a specified value from the list.
my_list = [1, 2, 3, 4, 2]
my_list.remove(2) # Removes the first occurrence of 2, the list becomes [1, 3, 4, 2]
5. pop()
: Removes and returns an item at a specified index (default is the last item).
my_list = [1, 2, 3, 4]
popped_item = my_list.pop(2) # Removes and returns the item at index 2 (3)
6. extend()
or +=
: Appends the elements of another iterable (e.g., another list) to the end of the list.
my_list = [1, 2, 3]
other_list = [4, 5]
my_list.extend(other_list) # Appends [4, 5] to the end of my_list
# Alternatively: my_list += other_list
7. index()
: Returns the index of the first occurrence of a specified value in the list.
my_list = [1, 2, 3, 4, 2]
index = my_list.index(2) # Returns the index of the first occurrence of 2 (1)
8. count()
: Returns the number of times a specified value appears in the list.
my_list = [1, 2, 3, 4, 2]
count = my_list.count(2) # Returns 2, as 2 appears twice in the list
9. sort()
: Sorts the list in ascending order (in-place).
my_list = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
my_list.sort() # Sorts the list in-place: [1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9]
10. reverse()
: Reverses the order of elements in the list (in-place).
my_list = [1, 2, 3, 4, 5]
my_list.reverse() # Reverses the list in-place: [5, 4, 3, 2, 1]
These are some of the fundamental functions and methods for working with lists in Python. Lists are powerful data structures that can be used for various purposes, and understanding these functions and methods is essential for effective list manipulation.